I had a situation recently where a client needed some custom validation logic during the submission of a form on their Umbraco v8 site. Since the site used the UmbracoForms Add-On I needed to hook into the form’s event pipeline and evaluate the contents of the form, then ensure the submitted data met certain criteria. I also needed to format some of the data before save, which I’ll discuss in another post on creating an Umbraco Forms Workflow.
In order to accomplish this task we’ll need to create an Umbraco Composer class. This should go into your custom code library. If you don’t have one setup, follow my guide in how I setup an Umbraco v8 project. So create a class, FormEventsComposer.cs
in your Project.Core/Composers/ folder.
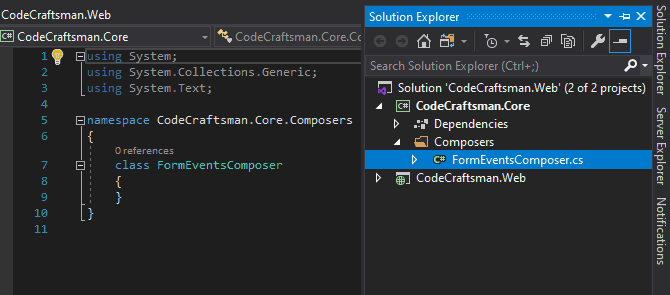
UmbracoForms has a global controller event that fires when a form has been submitted, but before it’s accepted. You can create a handler for this event and place any custom validation logic there. Wire it up within the constructor like so:
public void Compose(Composition composition)
{
Umbraco.Forms.Web.Controllers.UmbracoFormsController.FormValidate +=
UmbracoFormsController_FormValidate;
}
Allow VS to auto-generate the handler method, or paste from mine below. I’ve also added in a check to isolate the validation to a specific form by its friendly name.
private void UmbracoFormsController_FormValidate(object sender, Umbraco.Forms.Mvc.FormValidationEventArgs e)
{
if (e.Form.Name == "Application Form")
{
var controller = sender as Umbraco.Forms.Web.Controllers.UmbracoFormsController;
if (controller == null || !controller.ModelState.IsValid)
return;
// Validation logic here
}
}
I have a couple helper methods that make reading the posted field data a bit easier, so paste these in:
private static string GetPostFieldValue(Umbraco.Forms.Mvc.FormValidationEventArgs e, string key)
{
var field = GetPostField(e, key);
var value = e.Context.Request[field.Id.ToString()] ?? "";
return value;
}
private static Umbraco.Forms.Core.Models.Field GetPostField(Umbraco.Forms.Mvc.FormValidationEventArgs e, string key)
{
return e.Form.AllFields.SingleOrDefault(f => f.Alias == key);
}
Now just add your logic to validate the posted form values. As an example, I’ve just read in a fictitious Account Number field and validated the length. You can then cause the form to return an error by using the controller’s ModelState.AddModelError()
method.
private void UmbracoFormsController_FormValidate(object sender, Umbraco.Forms.Mvc.FormValidationEventArgs e)
{
if (e.Form.Name == "Application Form")
{
var controller = sender as Umbraco.Forms.Web.Controllers.UmbracoFormsController;
if (controller == null || !controller.ModelState.IsValid)
return;
var accountNumber = GetPostFieldValue(e, "accountNum");
if (accountNumber.Length < 10)
controller.ModelState.AddModelError(GetPostField(e, "accountNum").Id.ToString(), "Account number invalid!");
}
}
That’s it. You now have server-side validation of your UmbracoForm.