There’s a lot of posts out there describing how to force your own price to display when WooCommerce renders a product. Many of them are outdated or don’t handle all needed situations. The technique I’m sharing below is based on:
WooCommerce Version 6.9.4
These filters and actions will affect simple and variable products on the category page, product detail page, cart page, and mini-cart view. My hooks use a fixed price, but you can insert any custom logic needed into your own implementation.
I setup a visual example, first showing a typical simple and variable product on an archive/category page:

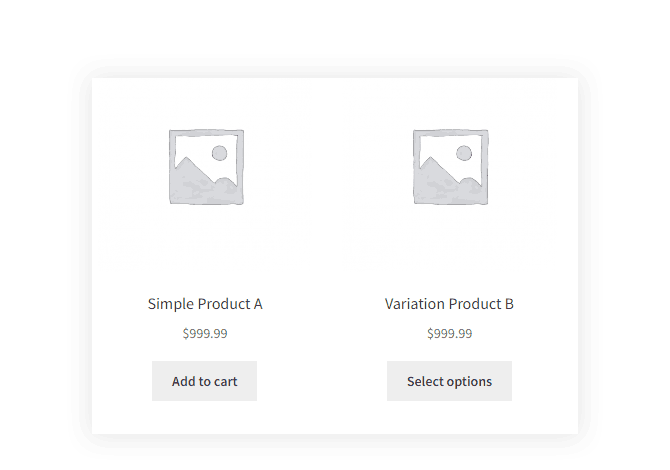
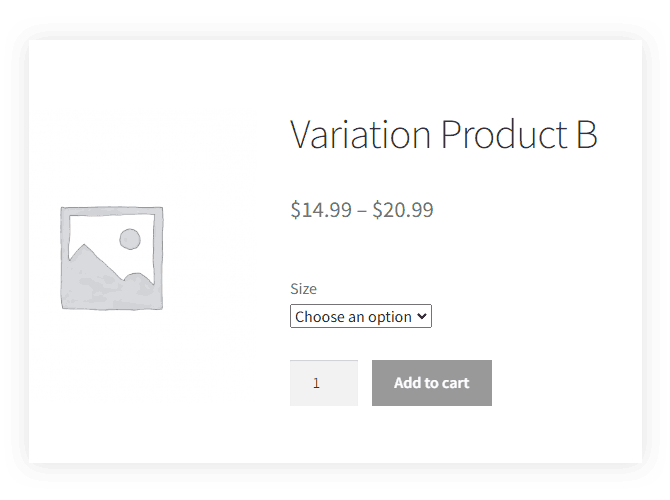

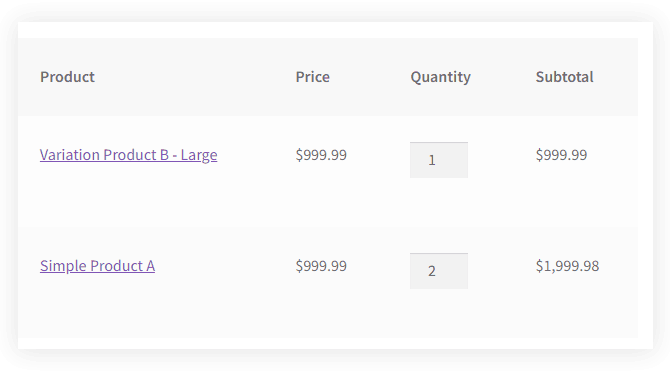
Implementing the price override
To achieve this level of control over your product pricing implement these filters and actions in your functions.php file or custom class:
function maybe_alter_product_price_excluding_tax( $price, $qty, $product ){
$price = 999.99;
return $price;
}
function maybe_alter_product_price( $price, $product ){
$price = 999.99;
return $price;
}
function maybe_alter_cart_item_data( $cart_item_data, $product_id, $variation_id ){
$cart_item_data['altered_price'] = 999.99;
return $cart_item_data;
}
function maybe_alter_calculate_totals( $cart_obj ) {
if ( is_admin() && ! defined( 'DOING_AJAX' ) )
return;
foreach ( $cart_obj->get_cart() as $key=>$value ) {
if ( isset( $value['altered_price'] ) ) {
$value['data']->set_price( $value['altered_price'] );
}
}
}
add_filter( 'woocommerce_product_get_price', 'maybe_alter_product_price', 99, 2 );
add_filter( 'woocommerce_get_price_excluding_tax', 'maybe_alter_product_price_excluding_tax', 99, 3 );
add_filter( 'woocommerce_variation_prices_price', 'maybe_alter_product_price', 99, 2 );
add_filter( 'woocommerce_add_cart_item_data', 'maybe_alter_cart_item_data', 99, 3 );
add_action( 'woocommerce_before_calculate_totals', 'maybe_alter_calculate_totals', 99, 1 );